One element mesh can not be drawn either with netgen.webgui.Draw or ngsolve.webgui.Draw.
from netgen.csg import Pnt
from netgen.meshing import Element3D, Element2D, MeshPoint, FaceDescriptor, Mesh
mesh = Mesh()
mesh.dim = 3
dom = {}
dom[1] = mesh.Add(FaceDescriptor(bc=1,domin=1,surfnr=1))
v = []
v.append(mesh.Add(MeshPoint(Pnt(0,0,0))))
v.append(mesh.Add(MeshPoint(Pnt(1,0,0))))
v.append(mesh.Add(MeshPoint(Pnt(0,1,0))))
v.append(mesh.Add(MeshPoint(Pnt(0,0,1))))
mesh.Add(Element3D(dom[1], v))
from netgen.webgui import Draw
Draw(mesh)
import ngsolve
mesh = ngsolve.Mesh(mesh)
from ngsolve.webgui import Draw
Draw(mesh)
Dof’s of the element seems to be ok, so the problem is Draw.
from ngsolve import *
print("norder, n_dof_curl_type1, n_dof_curl_type2, n_dof_H1")
for n in range(6):
fes_Curl_type1 = HCurl(mesh, order=n, type1=True)
fes_Curl_type2 = HCurl(mesh, order=n, type1=False)
fes_H1 = H1(mesh, order=n)
print([n, fes_Curl_type1.ndof, fes_Curl_type2.ndof, fes_H1.ndof])
use Element2D
mesh.Add(Element2D(dom[1], v))
and it will be drawn if “Mesh” is selected in the GUI. Not at “Geometry”.
I commented out Element3D and changed it to the following.
It is now the surface mesh and DoF’s have been decreased.
from netgen.csg import Pnt
from netgen.meshing import Element3D, Element2D, MeshPoint, FaceDescriptor, Mesh
mesh = Mesh()
mesh.dim = 3
dom = {}
dom[1] = mesh.Add(FaceDescriptor(bc=1,domin=1,surfnr=1))
v = []
v.append(mesh.Add(MeshPoint(Pnt(0,0,0))))
v.append(mesh.Add(MeshPoint(Pnt(1,0,0))))
v.append(mesh.Add(MeshPoint(Pnt(0,1,0))))
v.append(mesh.Add(MeshPoint(Pnt(0,0,1))))
mesh.Add(Element2D(dom[1], [v[0],v[1],v[2]]))
mesh.Add(Element2D(dom[1], [v[0],v[1],v[3]]))
mesh.Add(Element2D(dom[1], [v[1],v[2],v[3]]))
mesh.Add(Element2D(dom[1], [v[2],v[0],v[3]]))
#mesh.Add(Element3D(dom[1], v))
from netgen.webgui import Draw
Draw(mesh)
import ngsolve
mesh = ngsolve.Mesh(mesh)
from ngsolve.webgui import Draw
Draw(mesh)
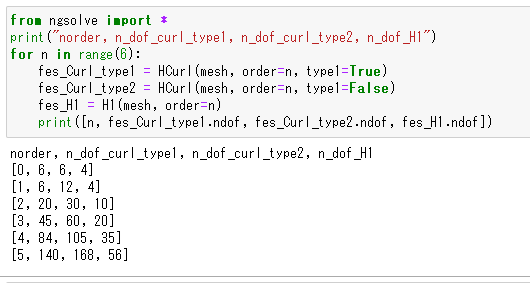
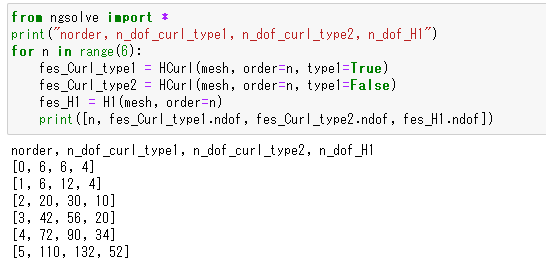
webgui currently doesn’t draw volume elements but only the surface element ones. But you can draw functions on the clipping plane of volume elements
In the usual fem applications you need both, volume elements and boundary elements.
E.g. if you do shells, or boundary element methods, only 2D Surface elements are enough.
If you add only volume elements, you cannot set boundary conditions (and you don’t get the surface visualization)
Joachim
I will use both Element2D and Element3D. For visualization, I understand Element2D is required.